A one-liner to generate passwords with PowerShell. Specify the length and the characters that build up the passwords and off you go!
We pick the characters that are picked using their ASCII codes. For example, numbers between 48 and 57 represent the ASCII code of the decimal numbers, 65 - 90 are the uppercase, and 97 - 122 are the lowercase letters of the English alphabet. 36 and 33, the $ and ! characters respectively, we use them as non-alphanumeric characters to make our passwords a little more complex.
You can cherry-pick your own character combinations as you like. Simply amend the script accordingly.
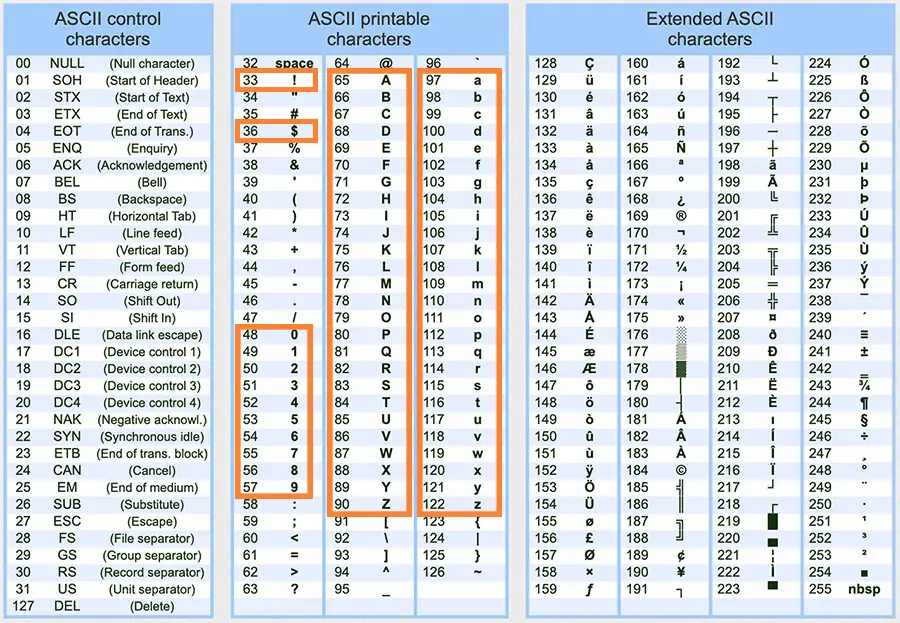
Method 1 - Create Passwords Manually with PowerShell
# Password length $length = 20 # Characters $chars = (48..57) + (65..90) + (97..122) + 36 + 33 # Generate 5 passwords for ($i = 1; $i -le 5; $i++) { [string]$Password = $null $chars | Get-Random -Count $length | %{ $Password += [char]$_ } # Print them out on the monitor Write-Host "Pass${i}: $Password" }
Method 2 - Use the DotNet Framework
# Generate a 20 character long random password, with at least 3 non-alphanumeric characters $Password = [System.Web.Security.Membership]::GeneratePassword(20, 3)
Example: Create a New User with a Random Password
$Password = [System.Web.Security.Membership]::GeneratePassword(20, 5) New-ADUser -Name "Test User" -Enabled $true -AccountPassword (ConvertTo-SecureString -AsPlainText "$Password" -Force)
Example2: Set Alice's AD Password
Set-ADAccountPassword "Alice" -NewPassword (ConvertTo-SecureString -AsPlainText "$Password" -Force)
Comments