Math in PowerShell is very simple and straightforward, the basic operators work as they usually do in any programming or scripting language: 1 + 1, 64 - 16, 4 * 32, 1024 / 128, etc.
However, many times I faced a conversion issue: converting bytes to KB, MB, GB … can be tedious.
For instance, say we query the sizes of mailboxes in Office365, after parsing the output we have very long numbers in bytes as the output. For instance:
We want to convert them into GB so it is easier to interpret for the human eye.
Now we could divide the byte value by 1024 three times, like: $GB = $ByteSize/1024/1024/1024, however it is not too elegant. We naturally expect to use a simple operator for the process, like (1024^3) in bash, or (1024 ** 3) in Python. PowerShell turns out to be very similar to C++ and Java in this case, we need to use the pow math operator to accomplish the task:
So let’s see how we convert the byte values quickly in an example:
$mbSizeInBytes = (
3612756732657,
32866565123645,
847757834758,
3845865824
)
$mbSizeInBytes | %{
$mbSizeInGB = $_/[math]::pow(1024, 3)
Write-Host "Mailbox size is: $mbSizeInGB GB !"
}
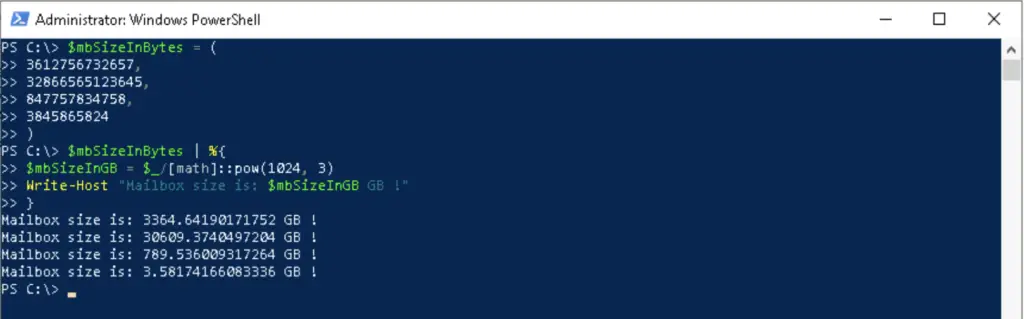
Comments