Do you want to automate distribution group creation, making your job easier? With this script you'll be able to mass-create distribution groups. It takes a simple text file as an input that contains a list of delivery group names, and members. The input list should look like this:
New Group Name
First Member
Second Member
Second Group Name
First Member
Second Member
Third Member
...
The blocks are separated with one or more new lines. Then the script check if the users exist or are unique in the system. If not, a detailed warning is thrown back.
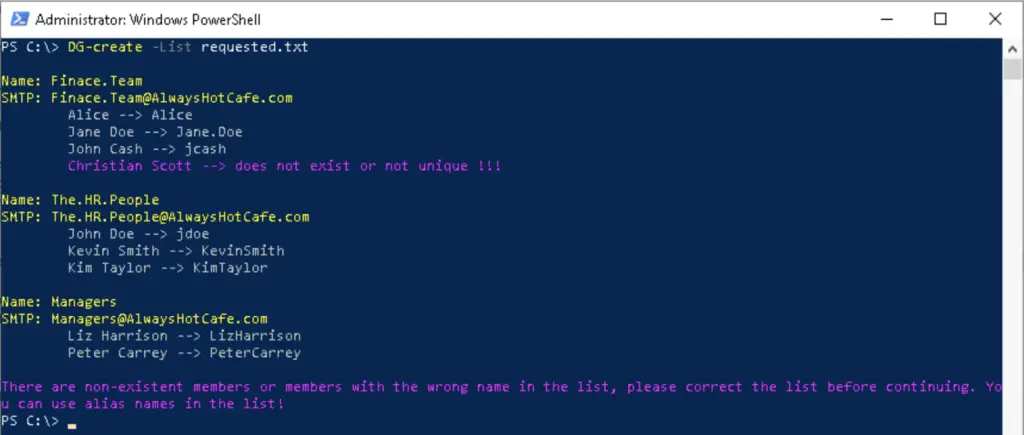
The function takes multiple arguments like the input file (if not specified it's dg.txt), also logging can be enabled. By default, the script runs dry, to actually create the groups use the -Commit switch!
DG-create -List dg.txt -ExternalAccepted:$true -Commit
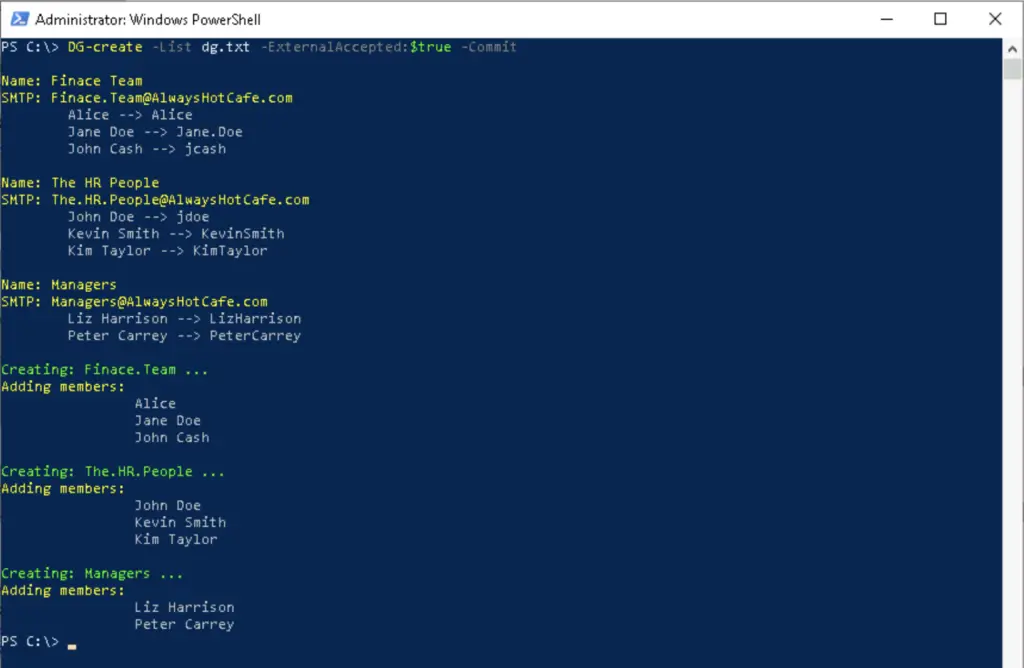
The Script
# Script to create delivery groups from file that consists of blocks devided by one or more empty lines. # The first entry of each block is the name of the delivery group, the subsequent entries are members with fullaccess- # and SendOnBehalf rights over it. The name can contain spaces, that case the script will replace them with a "." # # Example: DG-create -List requested.txt -Ticket WO000007684957 -Logging -Commit # # Parameters: # -List [file]: the location of the list file. If not defined, dg.txt by default # -Logging: if used, logging will be enabled and a log file is created in the current location # logfile name: DG-Create_yyy_MM_dd_HH_mm_ss.log # -ExternalAccepted: if used, external emails will be allowed. Default is InternalOnly # -Ticket: the WO number if exist # -Commit: use the switch to commit changes, otherwise a dry-run is performed # # # Example: # # New Group Name # First Member # Second Member # # Second Group Name # First Member # Second Member # ... # # Procedure: # 1. the script is checking if the members exist and are unique # 2. creating DG # 4. adding members to the groups function DG-create { param ($List, $Ticket, [switch]$Logging, [switch]$ExternalAccepted, [switch]$Commit) # Set logfile and the logging function, we use WriteLog to replace Write-Host in the rest of the script $logfile = "DG-Create_$((get-date).tostring("yyy_MM_dd_HH_mm_ss")).log" function WriteLog ($disp, $dispattr){ if (!($dispattr)) {$dispattr = "white"} write-host -f $dispattr $disp if ($Logging) {add-content $logfile -value $disp} } # Set and check if the list file exists if ($List -eq $null) {$listfile = "dg.txt"} else { $listfile = $List } if (!(Test-Path $listfile)) { write-host -n -f magenta "The list file does not exist: " write-host -f yellow "$listfile" break } # Get default domain $DefaultDomain = (Get-AcceptedDomain | ?{$_.default -eq $true}).domainname # Declare counters and array $i = $e = $dtaken = 0 $all = @{} # Building hash table with group - member association get-content $listfile | %{ if ($_ -like ""){ $i = 0 } else { if ($i -eq 0){ $i++ $dg = $_.trim().replace(" ",".") if ( !$all.$dg ) { $all.$dg = @() } } else { $all.$dg += $_.trim() } } } # Check and confirm groups and members foreach ($dg in $all.Keys) { # Check if the delivery group SMTP is already used if ((get-recipient "$dg@$DefaultDomain" -ErrorAction Silent) -eq $null) { write-host -f yellow "`r`nName: $($dg.replace("."," "))`r`nSMTP: $dg@$DefaultDomain" } else { write-host -f magenta "`r`nName: $dg`r`nSMTP: $dg@$DefaultDomain`t<== already used!!!" $dtaken++ } $all.$dg | %{ # Check if member exists or is unique. If unique, their alias names are displayed on the screen if ( (($mb = get-mailbox $($_).trim() -erroraction silent )| measure).count -eq 1) { write-host -f gray "`t$($_) --> $($mb.alias)" } else { $e++; write-host -f magenta "`t$($_) --> does not exist or not unique !!!"} } } # Display error check results if ($dtaken -ne 0) { write-host -f magenta "`r`nOne or more gelivery group address is already being used, please see above!" break} if ($e -ne 0) { write-host -f magenta "`r`nThere are non-existent members or members with the wrong name in the list, please correct the list before continuing. You can use alias names in the list!" break} if (!($Commit)) { write-host -f green "`r`nIf the above looks OK, please use the -Commit switch to proceed with the changes." break } #Create DGs foreach ($dg in $all.Keys) { $dg = $dg.trim() $dgDisplayed = $dg.replace("."," ") add-content $logfile -value "Time: $(get-date)" WriteLog "`r`nCreating: $dg ..." "green" New-DistributionGroup -Name $dgDisplayed -DisplayName $dgDisplayed -Alias $dg -PrimarySmtpAddress "$dg@$DefaultDomain" -RequireSenderAuthenticationEnabled $(!($ExternalAccepted)) -OrganizationalUnit "CN=Users,DC=AlwaysHotCafe,DC=com" | out-null Get-ADGroup -Filter {name -like "$dg"} | Set-ADGroup -Description "$Ticket" add-content $logfile -value "*****" WriteLog "Adding members:" "yellow" $all.$dg | %{ $dgmember = $_.trim() Add-DistributionGroupMember $dg -Member $dgmember | out-null WriteLog "`t`t$dgmember" "gray" } add-content $logfile -value "*****`r`n*****" } }
Comments